@danielp thank you for that long answer. I already started with learning more about that through reading.
@thoced thank you also, for your info but the pathfinding is one of the implemented functionalities, which works better than expected, It runs with A* too,
I have another question related to the GeometryComparator. To give you a better overview, here is a picture which should explain my issue;
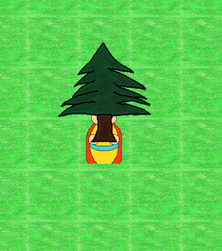
The geometry of my npc has a lower y coordinate than the tree. So the npc geometry should lay above the tree, so it seems like “3D”. I think you know what I mean. When the npc is behind the tree, so the y coordinate of the npc is higher than the y of the tree, then the tree should lay above. To solve that I wanted to experiment with the RenderQueue and therefor I tried to implemented my own YComparator like this:
public class YComparator implements GeometryComparator {
private Camera cam;
@Override
public void setCamera(Camera camera) {
this.cam = camera;
}
@Override
public int compare(Geometry o1, Geometry o2) {
if(o1.getLocalTranslation().y < o2.getLocalTranslation().y){
return -1;
} else {
return 1;
}
}
Now I don’t have any clue how to attach the new GeometryComparator to jMonkeyEngine, especially to this RenderQueue.Bucket. I found those calls but I do not know how to use them:
RenderQueue renderQueue = viewPort.getQueue();
renderQueue.setGeometryComparator(RenderQueue.Bucket.XYZ, new YComparator());
Additionaly I do not understand how I could attach my custom geometry to the objects where I want to use them. I found this call:
geo.setQueueBucket(RenderQueue.Bucket.XYZ);
Of course I know XYZ stands for e.g. Transparent or Opaque, but how can I use them with custom GeometryComparators and how can I attach them to special objects, like trees or npcs, I want to use them with. All the tiles in my tilemap, of course, mustn’t have this GeometryComparator.